Learn how to add a custom dropdown field in your widget from which you can select users.
In this tutorial, you will learn how to add a custom dropdown field, with a list of users to select from, to your custom widget.
This tutorial is for advanced developers.
You must have already created a custom widget before proceeding with this tutorial.
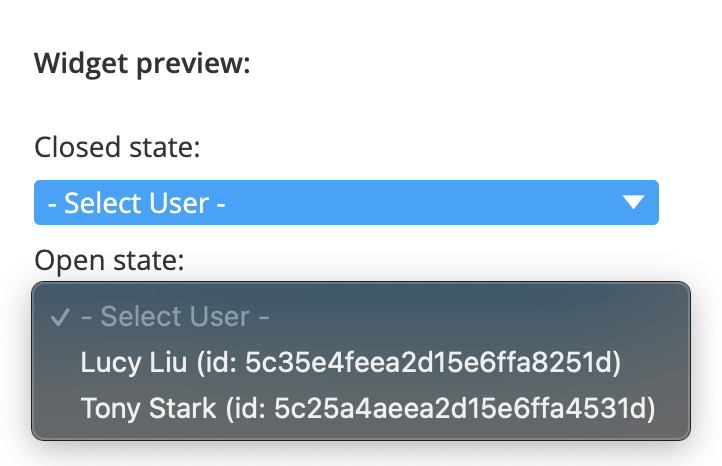
On completing this tutorial, you will be familiar with the properties and functions required to fetch users in order to display them in a dropdown field.
- Pass the widget API element as a property to your custom widget component.
1const factory = (Base, widgetApi) => {2 return class CustomBlock extends BaseBlockClass implements BaseBlock {3 constructor() {4 super();5 }6 ...7 renderBlock(container): void {8 ReactDOM.render(9 <CustomWidget {...this.props} widgetApi={widgetApi} />, container10 );11 }12 ...13 };14};
- Fetch users of your Staffbase App using the following function:
getUserList()
The following parameters are supported for getUserList() function:
- limit number
- offset number
- filter string
- sort enum: lastname, created, updated
- To display users in a dropdown list, use the following code snippet:
1import React, { useEffect, useState, FunctionComponent, ReactElement } from "react";2import { UserListItem, WidgetApi } from "widget-sdk";34export interface CustomWidgetProps {5 widgetApi: WidgetApi;6}78export const CustomWidget: FunctionComponent<CustomWidgetProps> = ({ widgetApi }: CustomWidgetProps): ReactElement | null => {910 const [users, setUsers] = useState<UserListItem[] | null>(null);1112 useEffect(() => {13 widgetApi.getUserList({ limit: 5 }).then((users) => {14 setUsers(users.data);15 });16 }, []);1718 return users ? (19 <div style={{ marginTop: 10 }} className="users-dropdown">20 <label htmlFor="users">21 {"User: "}22 <select name="users" id="users" defaultValue="none">23 <option value="none" disabled>24 - Select User -25 </option>26 {users.map((user) => (27 <option28 key={user.id}29 value={user.id}30 >{`${user.firstName} ${user.lastName}`}</option>31 ))}32 </select>33 </label>34 </div>35 ) : null;36};
You have added user dropdown field to your custom widget.