Learn how to apply the Staffbase App or Intranet theme to your custom widget.
Aligning your custom widget to the style and theme of your App or Intranet gives brand consistency and your users the feeling that your custom widget is a part of their app and intranet. In this tutorial, you will learn how to align your custom widget to the same color scheme used in your App or Intranet.
This tutorial is for advanced developers.
You must have already created a custom widget before proceeding with this tutorial.
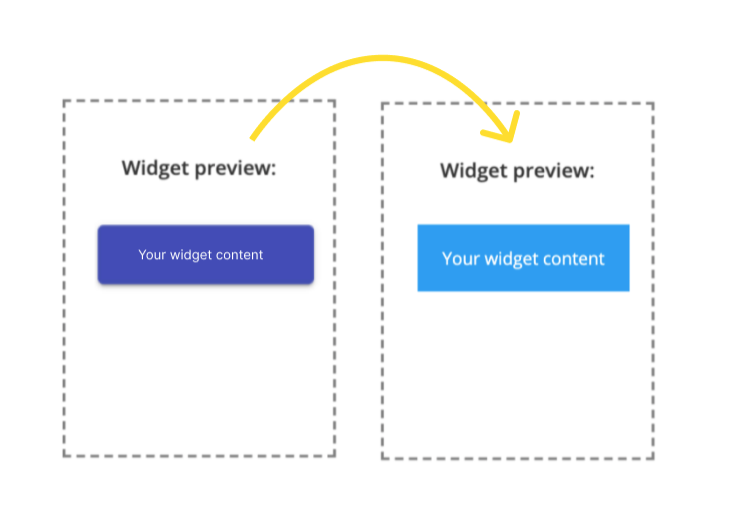
On completing this tutorial, you will be familiar with the properties and functions required to align with the text and the background color of your Staffbase App or Intranet.
- Pass the widget API element as a property to your custom widget component.
1const factory = (Base, widgetApi) => {2 return class CustomBlock extends BaseBlockClass implements BaseBlock {3 constructor() {4 super();5 }6 ...7 renderBlock(container): void {8 ReactDOM.render(9 <CustomWidget {...this.props} widgetApi={widgetApi} />, container10 );11 }12 ...13 };14};
For more information on branding, read branding for Staffbase App / Intranet.
- Apply the text and background color of the Staffbase App / Intranet to the main
div
element of your custom widget.
As this tutorial is designed to help you get started applying theme of your app or intranet, only changing text and background color is shown here. You can use this as a base and customize your widget further.
1import React, { useEffect, useState, FunctionComponent, ReactElement } from "react";2import { WidgetApi, ColorTheme } from "widget-sdk";34export interface CustomWidgetProps {5 widgetApi: WidgetApi;6}78export const CustomWidget: FunctionComponent<CustomWidgetProps> = ({ widgetApi }: CustomWidgetProps): ReactElement => {910 const [theme, setTheme] = useState<ColorTheme | null>(null);1112 useEffect(() => {13 setTheme(widgetApi.getLegacyAppTheme());14 }, []);1516 return <>17 <div style={{18 color: theme?.textColor,19 backgroundColor: theme?.bgColor,20 padding: "20px"21 }}>22 <div>Your widget content</div>23 </div>24 </>25};26
You have applied Staffbase App or Intranet text and background color to your custom widget.