Learn how to configure your custom widget to display the user information for different use cases.
The Custom Widget SDK allows you to configure your custom widget to display various user information based on your business use cases.
This tutorial is for advanced developers.
You must have already created a custom widget before proceeding with this tutorial.
In this tutorial, you will learn to display the following in your custom widget:
- Information of the signed-in user
- Custom profile fields of the signed-in user
As this tutorial is designed to help you get started retrieving user information, only a simple use case is shown. You can use this as a base and customize your widget further based on your business use case.
You can configure your custom widget to display user information. Use one of the following functions to retrieve the current user information:
getUserInformation()
- returns the current user when no parameter is used
- use Staffbase User ID as parameter to fetch a specific user:
getUserInformation(userId)
getUserInformationByExternalId()
- use external ID of user as parameter to fetch a specific user:
getUserInformationByExternalId(externalId)
- use external ID of user as parameter to fetch a specific user:
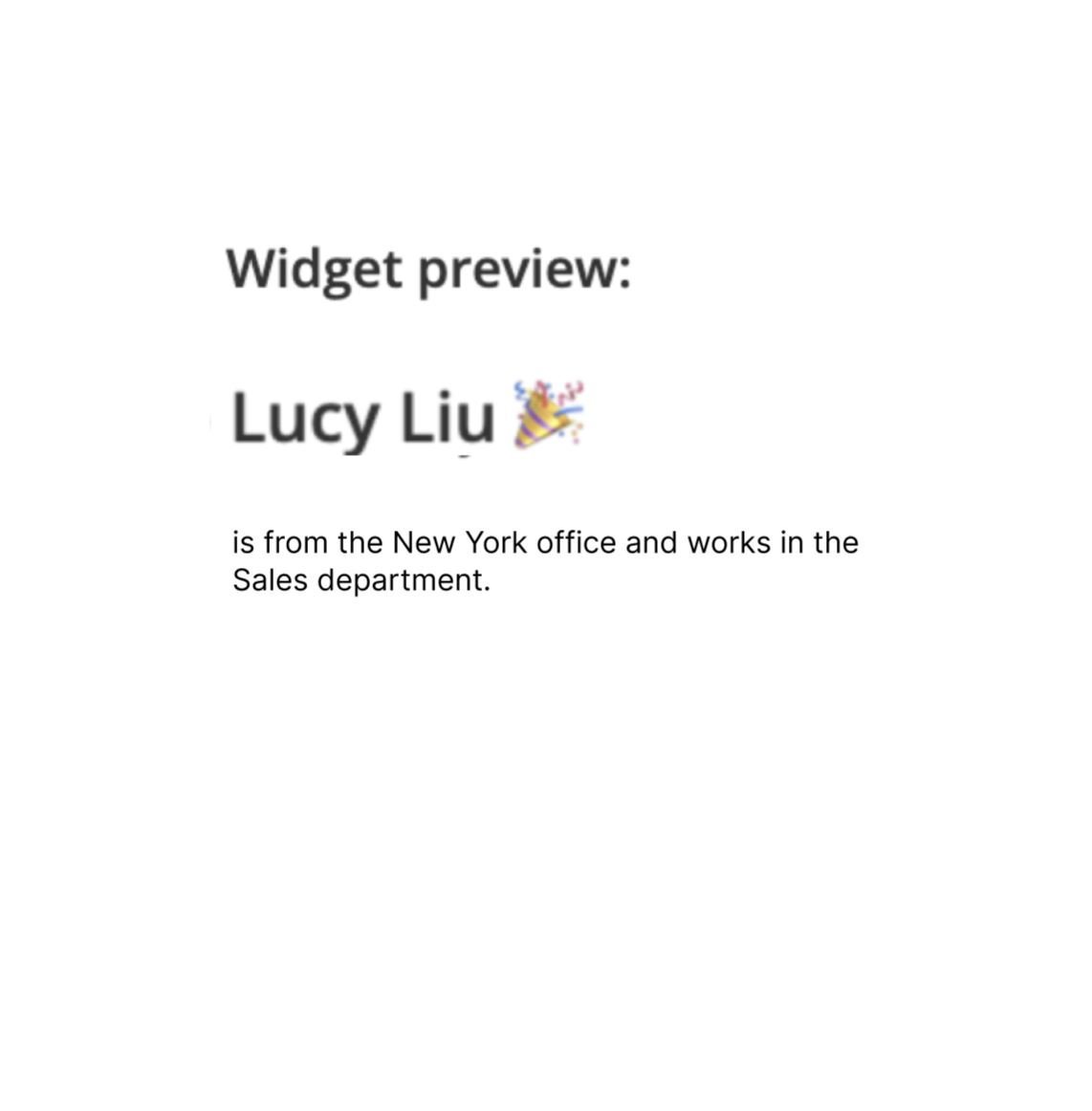
On completing these steps, you will be familiar with the properties and functions required to fetch user information.
- Pass the widget API element as a property to your custom widget component.
1const factory = (Base, widgetApi) => {2 return class CustomBlock extends BaseBlockClass implements BaseBlock {3 constructor() {4 super();5 }6 ...7 renderBlock(container): void {8 ReactDOM.render(9 <CustomWidget {...this.props} widgetApi={widgetApi} />, container10 );11 }12 ...13 };14};
The following user attributes are fetched:
- avatar
- department
- externalId
- firstName
- id
- lastName
- location
- phoneNumber
- position
The attributes represent the system profile fields within the Staffbase App or Intranet.
Read more about the Staffbase User Model here and about the Staffbase User Identifier (external ID) here.
In addition to the above attributes, if you want the widget to display the attributes primaryEmail and primaryUsername, you need to reach out to your Customer Success Manager to activate the option for you.
- Fetch the current user accessing the custom widget and display the user's attributes.
1import React, { useEffect, useState, FunctionComponent, ReactElement } from "react";2import { SBUserProfile, WidgetApi } from "widget-sdk";34export interface CustomWidgetProps {5 widgetApi: WidgetApi;6}78export const CustomWidget: FunctionComponent<CustomWidgetProps> = ({ widgetApi }: CustomWidgetProps): ReactElement | null => {910 const [user, setUser] = useState<SBUserProfile | null>(null);1112 useEffect(() => {13 widgetApi.getUserInformation().then((user) => {14 setUser(user);15 });16 }, []);1718 return <>19 { user ?20 <div>21 <h1 style={{ marginBottom: 10 }}>22 {user.firstName} {user.lastName} 🎉23 </h1>24 <p>is from the {user.location} office and works in the {user.department} department.</p>25 </div>26 :27 null28 }29 </>30};
You have configured your widget to display user information.
You can also display custom profile fields of users in your widget by using the custom profile field ID as an attribute key, example {user.customProfileFieldId}
.
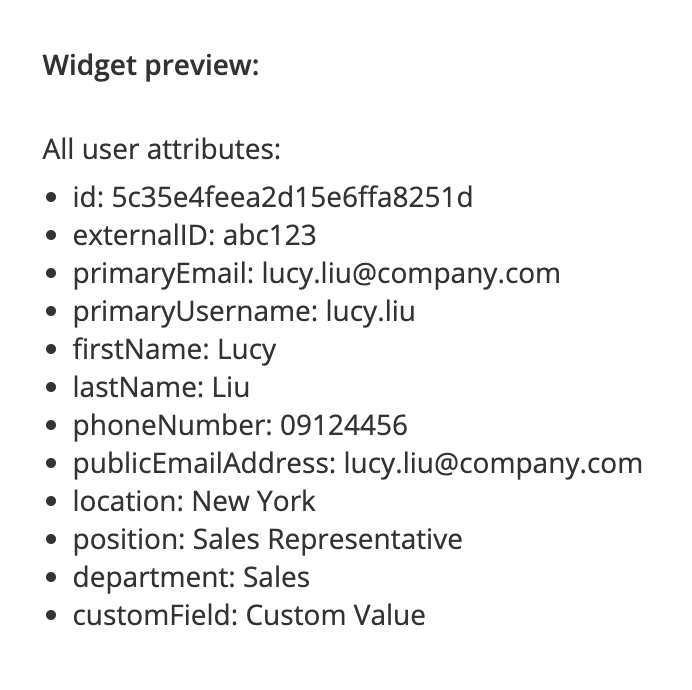
On completing these steps, you will be familiar with the properties and functions required to display custom profile fields of a user.
- Pass the widget API element as a property to your custom widget component.
1const factory = (Base, widgetApi) => {2 return class CustomBlock extends BaseBlockClass implements BaseBlock {3 constructor() {4 super();5 }6 ...7 renderBlock(container): void {8 ReactDOM.render(9 <CustomWidget {...this.props} widgetApi={widgetApi} />, container10 );11 }12 ...13 };14};
- Fetch custom profile fields.
1import React, { useEffect, useState, FunctionComponent, ReactElement } from "react";2import { SBUserProfile, WidgetApi } from "widget-sdk";34export interface CustomWidgetProps {5 widgetApi: WidgetApi;6}78export const CustomWidget: FunctionComponent<CustomWidgetProps> = ({ widgetApi }: CustomWidgetProps): ReactElement => {910 const [user, setUser] = React.useState<UserListItem[]>();1112 useEffect(() => {13 widgetApi.getUserInformation().then((info) => {14 setUser(info);15 });16 }, []);1718 return user ? (19 <div>20 <p style={{ marginBottom: 10 }}>All user attributes:</p>21 <ul>22 {Object.entries(user).map(([key, value]) => (23 <li>{`${key}: ${value}`}</li>24 ))}25 </ul>26 </div>27 ) : null;28};
You have configured your the custom widget to display custom fields.